MVC architecture is a very familiar term in the web development aspect. And, if you work in web development then, it is most likely that you have come across this acronym “MVC” a million times. It is one of the most famous design patterns in the world of web programming today.
So, in this article, we are going to discuss in detail the whole MVC architecture topic which includes the MVC basics, its advantages, and some code samples to understand this architecture better.
What is MVC Architecture Exactly?
Before we dive into the technical insights, let’s make it clear that MVC is not a design pattern; it’s a way that structures the web applications. Over the past few years, web applications are broadly adopting MVC architecture, and thus, people confuse it for a design pattern.
This term MVC was first described in 1979, even before the WWW era when the web applications didn’t exist. Earlier, this architecture was included in the two frameworks of web development i.e. Struts and Ruby on Rails.
For most of the web framework, Struts and Ruby on Rails environments laid the way hence, the popularity of MVC architecture kept growing.
MVC architecture follows a basic idea and its responsibilities are:
- Model: Business logic and handle data
- View: Whenever asked for data, presents the data to the user.
- Controller: Fetch the necessary resources and fulfills user requests.
Each component has a different set of tasks which ensures easy functioning of the whole application along with total modularity.
Let us now have a look at these components in detail.
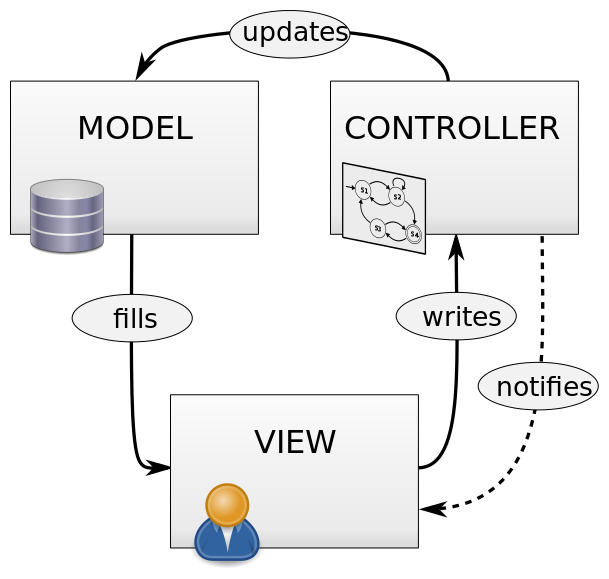
Model:
The model is simply the data for our application and this data is “modeled” in such a way that it is easy to store, edit, and retrieve. It also explains how rules are applied to the data. For any web application, everything is considered as data that is to be handled easily. This whole thing ultimately represents the concepts that are managed by the web application.
Think for an app, what is a user, or a message? It is just the data that is processed according to some set of rules. So, whenever a request is made from the controller, it contacts the concerned model which fetches some data representation. The model also contains the logic to update the appropriate controller if there is any change in the data of the model.
View:
The view is accountable for representing the data received via the model. For view there might be templates where the data can be fitted. And, depending on the requirements there might also be several views per model.
Controller:
The controller is just like a housekeeper of the web application, it performs synchronization between model and view to fulfill user requests. The requests from users are received as HTTP post or HTTP get request.
For example: To perform any action, the user clicks on any GUI elements and the primary purpose of a controller is to call and synchronize with the model to retrieve the necessary resources required to act. Generally, while receiving user requests, the controller calls a suitable model for the task.
A web application is always structured by keeping in mind these 3 core components. There may be a prime controller, responsible for receiving all the user requests and then calling the specific controller for specific actions.
Let’s now understand the workings of an application under the MVC architecture with an example.
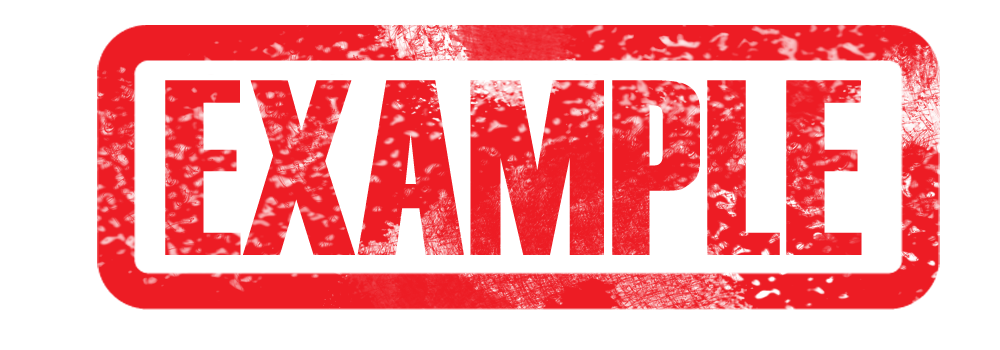
Example:
Suppose a user can view items, add items to the cart and buy items from an online stationery shop. So, now what happens when a user clicks on any item say-“Pencil” to view the list of pencils.
The application has a particular controller to handle all the requests related to the title pencil. Let’s assume “pencil_controller.php.” And, the model that would store the data related to the pencils “pencil_model.php.”
Then comes the views to stored data – a list of pencils, a table displaying pencils, and a page to edit the list.
The below-displayed figure shows the entire flow of control, from the moment a user select “pencil” till the results are retrieved:
The process starts with the “pencil_controller.php,” it handles the user request as an HTTP GET or HTTP POST request. There can also be a central controller – “index.php” to call the “pencil_controller” whenever needed.
Then the controller examines the request and the parameters. Then after, it calls the required model, considering the above case, “pencil_model.php” will be called. Then, the controller asks the model to fetch the list of available pencils.
Now, the model searches through the database for all the necessary information, implements the logic; if necessary, and retrieves the required data to the controller. Hence, the controller picks a suitable view and presents the data. In case, the request comes from a handheld device, a suitable view will be used accordingly.
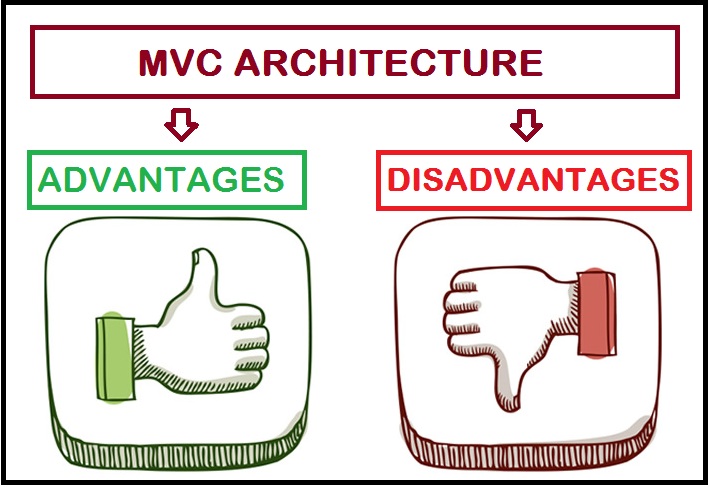
Advantages of MVC Architecture
1. Faster development process
MVC model supports parallel and rapid development. Also, in order to create the business logic of the web application, an MVC model is used in the development of a web application. And, it implies that one programmer would be working on the view while others would be working on the controller.
Hence, the application is developed in an MVC model is 3X faster than applications developed by using other development patterns.
2. Ability to provide multiple views
MVC model is used to create multiple views for a single model. In today’s world, an application is accessed in multiple ways and for this versatility MVC development is definitely a great solution.
Furthermore, in this method, duplication of code is very limited as it separates business logic and data from the display.
3. Support for asynchronous technique
The MVC model integrates with the JavaScript framework which means that the applications developed in MVC can work even with PDF files, site-specific browsers, and even with desktop widgets.
It also supports asynchronous technique that helps developers to build an application that can be loaded very fast.
4. Modification does not affect the entire model
The User interface of a web application tends to change more often than the business rules of the company. So, it is more likely that you’ll make changes in your web application e.g. changing colors, screen layouts, fonts, and adding new device support for smartphones frequently.
Moreover, adding new types of views is very easy in the MVC pattern because the Model part does not depend on the views part. Therefore, the entire architecture is not affected if any change is made in the Model.
5. MVC model returns the data without formatting
MVC model returns data without implementing any formatting. Thus, the same components could be called with any interface. For example, any type of data can be formatted with HTML, with Dream viewer or Macromedia Flash.
Disadvantages of MVC Architecture
1) More complexities
2) Difficulty in using MVC with the present-day user interface.
3) Inefficient data access in View component.
4) Requires multiple programmers
5) More knowledge needed on multiple technologies.
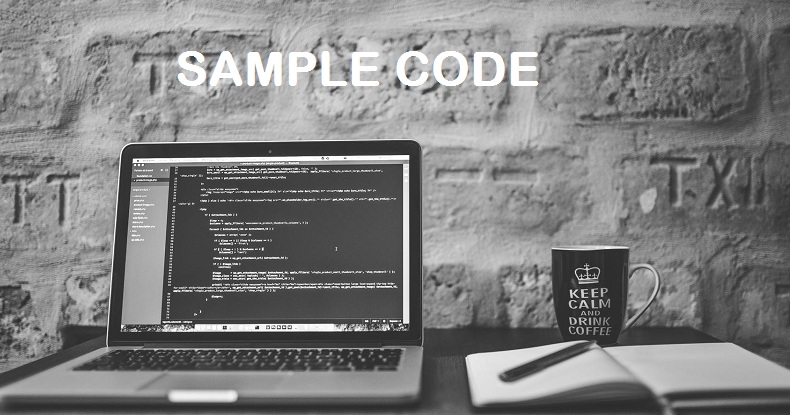
An Easy Illustration of MVC Architecture Using Java
1. Stu_Object: the model.
2. Stu_View: view class to print details on the console.
3. Stu_controller: a controller that stores data in Stu_Object and updates Stu_View accordingly.
Step 1: Creating the Model
public class StudentMVC {
private String Stu_id;
private String Stu_name;
public String getStu_id() {
return Stu_id;
}
public void setStu_id(String Stu_id) {
this.Stu_id = Stu_id;
}
public String getStu_name() {
return Stu_name;
}
public void setStu_name(String Stu_name) {
this.Stu_name = Stu_name;
}
}
The code consists of functions to get/set Stu_id and Stu_names of the StudentMVCs.
Let’s call it “StudentMVC.java”.
Step 2: Creating the View
public class Stu_View {
public void printStu_details(String StudentMVCStu_name, String StudentMVCStu_id){
System.out.println(“StudentMVC: “);
System.out.println(“Stu_name: ” + StudentMVCStu_name);
System.out.println(“Stu_id: ” + StudentMVCStu_id);
}
}
To print the values to the console. Let’s call this “Stu_View.java”.
Step 3: Create the Controller
public class Stu_controller {
private StudentMVC model;
private Stu_View view;
public Stu_controller(StudentMVC model, Stu_View view){
this.model = model;
this.view = view;
}
public void setStudentMVCStu_name(String Stu_name){
model.setStu_name(Stu_name);
}
public String getStudentMVCStu_name(){
return model.getStu_name();
}
public void setStudentMVCStu_id(String Stu_id){
model.setStu_id(Stu_id);
}
public String getStudentMVCStu_id(){
return model.getStu_id();
}
public void update_View(){
view.printStu_details(model.getStu_name(), model.getStu_id());
}
}
Call this “Stu_controller.java”. A cursory glance tells you that this controller is responsible for calling the model to get/set the data, and later updating the view.
Now, let’s have a look at how all of this is tied together.
Step 4: Creating the Main Java File
public class MVCPattern1 {
public static void main(String[] args) {
//fetch StudentMVC record based on his roll no from the database
StudentMVC model= retriveStudentMVCFromDatabase();
//Create a view: to write StudentMVC details on console
Stu_View view = new Stu_View();
Stu_controller controller = new Stu_controller(model, view);
controller.update_View();
//update model data
controller.setStudentMVCStu_name(“Johny”);
controller.update_View();
}
private static StudentMVC retriveStudentMVCFromDatabase(){
StudentMVC StudentMVC = new StudentMVC();
StudentMVC.setStu_name(“Rob”);
StudentMVC.setStu_id(“20”);
return StudentMVC;
}
}
This is called “MVCPattern1.java,” it fetches the StudentMVC data from the database or a function and pushes it on to the StudentMVC model.
After that, it initializes the view we had created earlier.
Also, it also initializes our controller & binds together it to the model and the view. The update_View() method is a part of the controller which updates the StudentMVC details on the console.
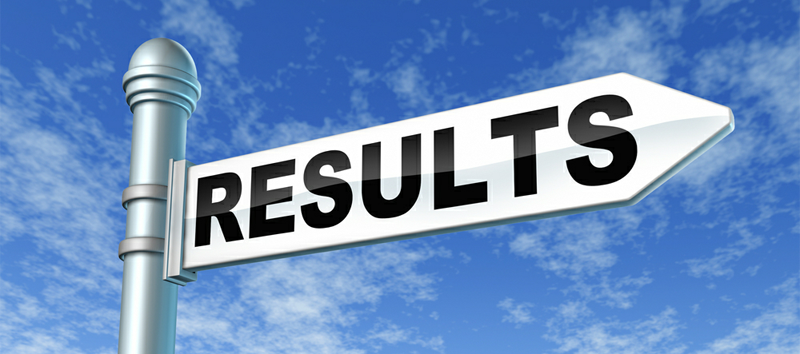
Step 5: Testing the Result
If everything goes right, the result should be:
StudentMVC:
Stu_name: Rob
Stu_id: 20
StudentMVC:
Stu_name: Johny
Stu_id: 20
If you get this output then, congratulations! You have successfully applied the MVC architecture using Java.
Conclusion
MVC is a way that structures the web applications and over the past few years, web applications are broadly adopting the MVC architecture, and thus, people confuse it for a design pattern. In case, you are looking for great web applications then, Orion eSolutions is famous for its exemplary IT services.